One of the biggest challenges that hardly anyone really talks about is getting hold of an existing project and not completely reprogramming it, but revising it on the fly. In this case, you don't have the luxury of planning an architecture from scratch. Who doesn't know it: All too often, the associated documentation is only helpful in places and former teams are just two steps out the door.
The following blog post is therefore not intended to shed light on the technical details of individual code implementations, but rather to provide an overview of how an existing project can be improved in a regulated manner and what thought processes underlie this process.
But where do you draw the line here? When is it worthwhile to continue working with an existing code base instead of starting with a clean sheet?
The moon landing: First contact with the project
When I was able to take a look at smartmove's web portal for the first time, I already saw a very solid core. They had obviously thought about logical modularization, and the associated routing had been implemented accordingly. It quickly became clear that know-how from the mobility sector had been incorporated here to define and define the individual sub-areas of the Angular project. Many features worked, the web app had already seen some customers and had done some work.
However, the first pain points also emerged quite quickly: The goal of a programmer should always be to develop code that is maintainable, scalable and as reusable as possible, but only too often do you find yourself confronted with a different reality in existing projects. My mentor a few years ago liked to call such repositories “organically grown”; in short, this means that the original, imaginary structure is still recognizable, but it is partly buried under a growth of bug fixes and decisions made at short notice.
At this point, it should be said that an experienced programmer should try not to make hasty judgments about existing code projects. All too often, the reasons behind some strange-looking structures in these “organically grown projects” are not easy to see, even when care was taken to leave in-line comments. The scripts, which seem opaque to us, may have been used in the past to meet obscure requirements that are unknown today. It is therefore not always the best idea to rip out entire script blocks and rewrite them, even if unraveling some components seems like a terrible Sisyphean task. All too often, in such a case, we risk disposing of old bug fixes.
If the schedule allows, I also always recommend tackling some small bug fixes. The aim here is not to improve the project, but rather the opportunity to gain a bit of an overview using a practical example. How does the code “flow” through the application, which coding patterns are repeated over and over again? Once you have developed a feeling for these questions, future clean-up work is much less nebulous.
Identify the biggest problem areas
As already mentioned above, my predecessors of the existing smartmove web portal project had already made sure that the compartmentalization was thematically appropriate. Areas such as vehicles, bookings and customer data were divided into their own useful modules.
Within these modules, however, at the hierarchical level of individual components and front-end services, there was a need for improvement in two points that could be explained quite roughly:
- Services
Even though an attempt was made to create singleton structures for higher-level procedures and functions (e.g. to handle different vehicle lists), a large amount of specific logic from different areas had apparently migrated into these singletons over time. The result was many inflated services that required a clear division of competencies.
- components
In the area of individual components, there was often a rather unclear flow of data, a fact that was further aggravated by the use of a third-party user interface solution. It was rarely really clear which components should deal with pure UI and in which areas the business logic was located. In many places, these two parts became blurred together, almost inextricably linked by code, which also had to ensure that the mentioned third-party UI solution was pressed into the appropriate shape.
Both of these topics seemed important, but the services at least did their job and rarely got in the way of new features. After just a short time, the insufficiently separated architecture at component level emerged as a major problem, especially against the background of the trinity of maintainability, scalability and reuse mentioned above.
The solution, part 1: The Facade pattern
After reviewing the problem areas listed in the last section, a fairly rapid brainstorming phase followed to evaluate possible solutions. After a short, successful test run using a new feature, the choice was made on an implementation based on the Facade Software Pattern. Explained in a very simple way, the Facade Pattern allows you to insert a kind of intermediate level that hides complex, often confusing code. This can be compared to the façade of a house, under which all kinds of struts, pipes and cables can lie.
A façade thus supports the separation of client facing, UI components that are as simple as possible (keyword: dumb components) and the business logic involved.
The clearly defined boundary of a façade made it significantly easier to subject the code of existing components to an initial division, but that alone was not enough.
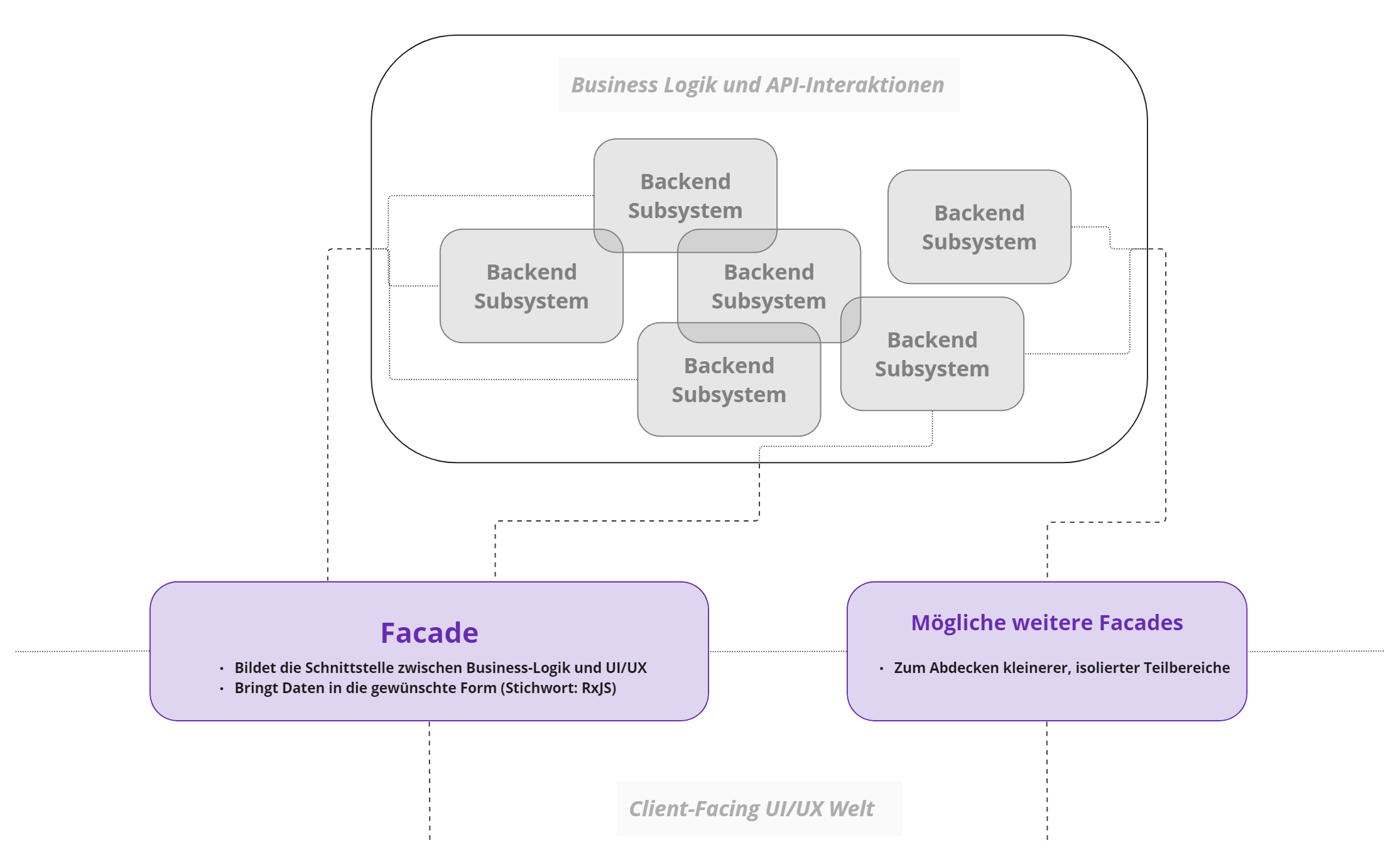
The solution, part 2: Smart and Dumb Components
In the course of the refactoring work, it became clear relatively quickly that facades alone would not deliver our desired result. When restructuring existing code, our focus was on simple, reusable components that, in the best case, should only be used to display user interfaces. But these components in particular should not interact directly with the implemented façade or ask for the required display data themselves.
It therefore required a single command center, a smart component for each feature, whose task was to interact with the façade. In addition, this wrapper component was responsible for composing the “stupid” UI components: Data should flow from the façade into the wrapper component, which in turn enveloped the individual UI parts and distributed the necessary data further down.
In this way, the area in front of the façade could be handled well, but where did the mentioned data come from? What happened to the code that was now hidden behind the façade?

The solution, part 3: buddy services
While a façade was used in the first attempt, as already described, to make complex code more easily accessible, the area behind the façade should then also be structured. Each façade received an additional small buddy service, which was to deal exclusively with backend/API interaction: The remote. The advantage of this remote was to provide a clear point of contact for back-end front-end interaction, which in turn made it easier to find possible bugs in this area. Remote access (and therefore to any API interactions) should only take place via the façade.
In addition, some features required the ability to request the status of their respective user interface and the underlying data accordingly. These features were therefore equipped with another buddy service for the façade: the State Management Service. This area was concerned with managing and managing a feature-specific UI model. Only here could data be changed and re-sent; in the rest of the feature structure, information was simply passed through and shaped, but never changed. By limiting data changes in this way, we were able to guarantee that no unwanted side effects were passed on to other areas of the code. Data now flowed from our State Management Service through the façade into the central wrapper component to be distributed to the underlying UI components. As a result of this structured architecture, every component that interacts with the façade via our central interface will always have access to the same, current instance of the UI state.
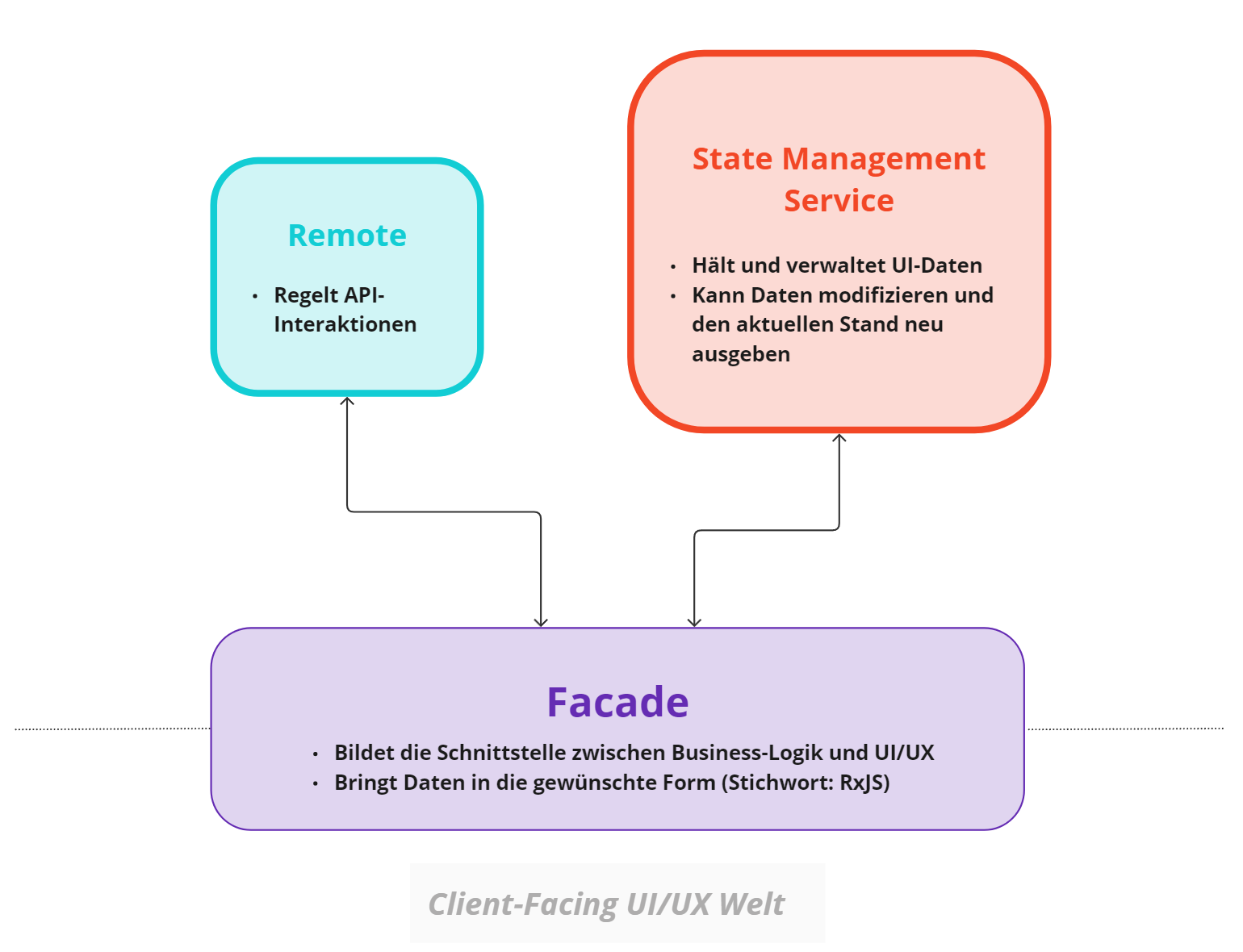
Bottom line: The work is never over
The implementation of the structure explained above quickly paid off:
- Maintaining and managing revised areas has become much easier - both for UI-specific bugs and for problems with business logic.
- The explained architecture formed a blueprint, a clear, comprehensible approach and for refactoring and sharing other existing features. The basic functions for managing the UI model were packaged into an abstract core to provide each feature-specific state management service with the same set of tools.
- Unwanted, UI-based side effects could be reduced to a minimum, as the current state of the user interface is managed by a state service.
- Since both revised and new features now followed the same, simple structure with clear data flow and core areas, it was much easier to find bugs and train more programmers.
At this point, however, it should be said that despite all these changes and improvements, not everything was done: As mentioned above, there were still some existing services that were suffering from the burden of their accumulated methods. A large area of the web portal was also still tied to that third-party UI solution, even though it was now only wreaking havoc in the clearly separated client-facing areas.
Our facade-based architecture was the first big step, because the clear division of the code made it easier to pull in and rework the now structured components piece by piece without risking a major, unwanted cascade effect.
If you want to think further, you can find out more about designing your own generalized, reusable UI components — the solution we use to be able to completely replace the third-party UI components available in the web portal.